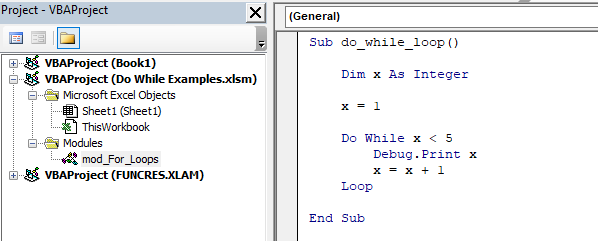
In VBA, a Do While loop is a type of loop that will continue to repeat a set of instructions until a specific condition is met.
Contents:
2. Example 1 - Do While x < 5
3. Example 2 - Do While String Not Equal to "x"
3. Exit Do Statement
How to Use Do While Loops in VBA
What are Do While Loops?
A Do While loop is a type of loop that will continue to repeat a set of instructions until a specific condition is met. This is different from a For loop, which will execute a set of instructions a specific number of times.
There are several reasons why you might want to use a Do While loop.
To repeat a set of instructions until a certain condition is met. For instance, you might use a Do While loop to continue prompting a user for input until they enter a valid response.
To repeat a set of instructions until a specific event occurs. For example, you might use a Do While loop to continue checking for new data from a sensor until data is received.
To repeat a set of instructions until a specific time or date is reached. For instance, you might use a Do While loop to continue running a simulation until a certain time or date is reached.
Do While loops are useful anytime a condition can be met early while looping, rather than repeating the instructions multiple times. This allows you to have more control over when the loop will stop running.
Example 1 - Do While "x" is Less Than "y"
Dim x As Integer
x = 1
Do While x < 5
Debug.Print x
x = x + 1
Loop
In this example, the loop will continue to run as long as the value of x is less than 5. Each time the loop runs, the value of x is printed to the debug window, and then x is incremented by 1. This means that the loop will run 4 times, printing the values 1, 2, 3, and 4 to the debug window before it is finished.
Example 2 - Do While String is Not Equal To "x"
Dim password As String
password = ""
Do While password <> "secret123"
password = InputBox("Please enter the password:")
If password = "secret123" Then
Debug.Print "Correct password. Access granted."
Else
Debug.Print "Incorrect password. Please try again."
End If
Loop
In this example, the loop will continue to run until the user enters the correct password. Each time the loop runs, it will prompt the user to enter the password, and then check if the entered password is correct. If the password is correct, a message is printed to the debug window, and the loop is finished. Otherwise, the loop will continue to run, prompting the user to enter the password again. This continues until the user enters the correct password.
Exit Do Statement
An 'Exit Do' statement can be used in to exit a Do loop early. This can be useful when a condition is met, and there is no need to continue looping.
Here is an example of how this might be used:
Do While condition = True
'Do something
If condition_secondary = True Then
Exit Do
End If
'Do something else
Loop
In this example, the Do While loop will continue to run as long as condition is True. However, if condition_secondary is also True, then the Exit Do statement will cause the loop to end, and execution will continue with the code after the Loop statement. This allows you to exit the loop early.